Hi,
I'm heaving problems with getting my triangle drawing routine to clip from the left and top edges. The right and bottom edges already work.
This is what it's doing:
After over a week I got a different result, but I don't really know which one is the closest to good clipping, but in this screenshot, I added 100 to the x coordinates before the calculating part of the routine, then substracting 100 again from the coordinates before it draws the pixels. This is what it looks like:
Here is the code of my triangle drawing routine (sorry, it isn't commented well and comments are in Dutch, and it's long too):
Code:
I'm heaving problems with getting my triangle drawing routine to clip from the left and top edges. The right and bottom edges already work.
This is what it's doing:
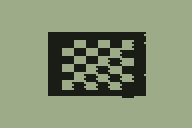
After over a week I got a different result, but I don't really know which one is the closest to good clipping, but in this screenshot, I added 100 to the x coordinates before the calculating part of the routine, then substracting 100 again from the coordinates before it draws the pixels. This is what it looks like:
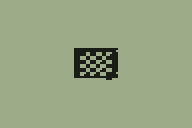
Here is the code of my triangle drawing routine (sorry, it isn't commented well and comments are in Dutch, and it's long too):
Code:
DrawTriangle:
;IN: x1,y1,u1,v1,x2,y2,u2,v2,x3,y3,u3,v3
;scherm = 96*64
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld de, 100
ld hl, (x1)
add hl, de
ld (x1), hl
ld hl, (x2)
add hl, de
ld (x2), hl
ld hl, (x3)
add hl, de
ld (x3), hl
;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld hl, (y1)
ld de, (y2)
cpHLDE
jr c, Y1SmallerThanY2
ld (y1), de
ld (y2), hl
ld hl, (x1)
ld de, (x2)
ld (x1), de
ld (x2), hl
#comment
ld hl, (u1)
ld de, (u2)
ld (u1), de
ld (u2), hl
#endComment
ld a, (u1)
ld b, a
ld a, (u2)
ld (u1), a
ld a, b
ld (u2), a
ld a, (v1)
ld b, a
ld a, (v2)
ld (v1), a
ld a, b
ld (v2), a
Y1SmallerThanY2:
ld hl, (y1)
ld de, (y3)
cpHLDE
jr c, Y1SmallerThanY3
ld (y1), de
ld (y3), hl
ld hl, (x1)
ld de, (x3)
ld (x1), de
ld (x3), hl
#comment
ld hl, (u1)
ld de, (u3)
ld (u1), de
ld (u3), hl
#endcomment
ld a, (u1)
ld b, a
ld a, (u3)
ld (u1), a
ld a, b
ld (u3), a
ld a, (v1)
ld b, a
ld a, (v3)
ld (v1), a
ld a, b
ld (v3), a
Y1SmallerThanY3:
ld hl, (y2)
ld de, (y3)
cpHLDE
jr c, Y2SmallerThanY3
ld (y2), de
ld (y3), hl
ld hl, (x2)
ld de, (x3)
ld (x2), de
ld (x3), hl
#comment
ld hl, (u2)
ld de, (u3)
ld (u2), de
ld (u3), hl
#endComment
ld a, (u2)
ld b, a
ld a, (u3)
ld (u2), a
ld a, b
ld (u3), a
ld a, (v2)
ld b, a
ld a, (v3)
ld (v2), a
ld a, b
ld (v3), a
Y2SmallerThanY3:
res 0, (IY) ;in bit 0, (IY) wordt opgeslagen bij welke helft het is. 0=eerste helft, 1= tweede helft
res 1, (IY) ;bit 1, (IY) = interval texturen al berekend
ld hl, (y2)
ld de, (y1)
subFP ;hier gebruikt om 16-bit getallen af te trekken I.P.V. fixed-point getallen
ld h, l
ld l, 0
push hl
ld hl, (x2)
ld de, (x1)
subFP
ld h, l
ld l, 0
pop de
call DivFP
ld (dx1), hl
ld hl, (y3)
ld de, (y2)
subFP
ld h, l
ld l, 0
push hl
ld hl, (x3)
ld de, (x2)
subFP
ld h, l
ld l, 0
pop de
call DivFP
ld (dx2), hl
ld hl, (y3)
ld de, (y1)
subFP
ld h, l
ld l, 0
push hl
ld hl, (x3)
ld de, (x1)
subFP
ld h, l
ld l, 0
pop de
call DivFP
ld (dx3), hl
ld hl, (y2)
ld de, (y1)
subFP
ld h, l
ld l, 0
push hl
ld a, (u2)
ld h, a
ld l, 0
ld a, (u1)
ld d, a
ld e, 0
subFP
;ld h, l
;ld l, 0
pop de
call DivFP
ld (du1), hl
ld hl, (y3)
ld de, (y2)
subFP
ld h, l
ld l, 0
push hl
ld a, (u3)
ld h, a
ld l, 0
ld a, (u2)
ld d, a
ld e, 0
subFP
;ld h, l
;ld l, 0
pop de
call DivFP
ld (du2), hl
ld hl, (y3)
ld de, (y1)
subFP
ld h, l
ld l, 0
push hl
ld a, (u3)
ld h, a
ld l, 0
ld a, (u1)
ld d, a
ld e, 0
subFP
;ld h, l
;ld l, 0
pop de
call DivFP
ld (du3), hl
ld hl, (y2)
ld de, (y1)
subFP
ld h, l
ld l, 0
push hl
ld a, (v2)
ld h, a
ld l, 0
ld a, (v1)
ld d, a
ld l, 0
subFP
;ld h, l
;ld l, 0
pop de
call DivFP
ld (dv1), hl
ld hl, (y3)
ld de, (y2)
subFP
ld h, l
ld l, 0
push hl
ld a, (v3)
ld h, a
ld l, 0
ld a, (v2)
ld d, a
ld e, 0
subFP
;ld h, l
;ld l, 0
pop de
call DivFP
ld (dv2), hl
ld hl, (y3)
ld de, (y1)
subFP
ld h, l
ld l, 0
push hl
ld a, (v3)
ld h, a
ld l, 0
ld a, (v1)
ld d, a
ld e, 0
subFP
;ld h, l
;ld l, 0
pop de
call DivFP
ld (dv3), hl
ld hl, (x1)
bit 7, h
jr z, TPos1
ld (tx1+1),hl \ ld a, $FF \ ld (tx1),a
ld (tx2+1),hl \ ld a, $FF \ ld (tx2),a
jr TEnd1
TPos1:
ld (tx1+1),hl \ xor a \ ld (tx1),a ;store the 16bit integer at hl into 16.8 fixed point number tx1
ld (tx2+1),hl \ xor a \ ld (tx2),a
TEnd1:
ld hl, (y1)
ld (_ty), hl
ld a, (u1)
ld h, a
ld l, 0
ld (tu1), hl
ld (tu2), hl
ld a, (v1)
ld h, a
ld l, 0
ld (tv1), hl
ld (tv2), hl
ld hl, (Y1)
ld de, (y2)
cpHLDE
jp z, __TEndLoop
TDrawLoop:
ld a, (_ty)
ld d, a
bit 7, a
jp nz, Clip
ld a, (_ty)
cp 64
ret nc
ld hl, (tu1)
ld (tmpu), hl
ld hl, (tv1)
ld (tmpv), hl
ld hl, (tu2)
ld (temp2), hl
ld hl, (tv2)
ld (temp3), hl
ld a, (tx2+1)
ld (temp+1), a
ld b, a
ld a, (tx1+1)
ld (temp), a
cp b
jr c, TOrdered
ld hl, (tu2)
ld (tmpu), hl
ld hl, (tv2)
ld (tmpv), hl
ld hl, (tu1)
ld (temp2), hl
ld hl, (tv1)
ld (temp3), hl
ld a, (tx2+1)
ld (temp), a
ld a, (tx1+1)
ld (temp+1), a
TOrdered:
ld l, d
ld a, (temp)
sub 100 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
call GetPixel
ld (mask), a
ld (pointer), hl
bit 1, (IY)
jr nz, TPlotLoop
ld hl, (tx1)
ld de, (tx2)
cpHLDE
jr z, TPlotLoop
ld a, (temp)
sub 100 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld h, a
ld l, 0
ld a, (temp+1)
sub 100 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld d, a
ld e, 0
subFP
push hl
ld hl, (tmpu)
ld de, (temp2)
subFP
pop de
call DivFP
ld (tmpdu), hl
ld a, (temp)
sub 100 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld h, a
ld l, 0
ld a, (temp+1)
sub 100 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld d, a
ld e, 0
subFP
push hl
ld hl, (tmpv)
ld de, (temp3)
subFP
pop de
call DivFP
ld (tmpdv), hl
set 1, (IY)
TPlotLoop:
ld a, (temp)
sub 100 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
bit 7, a
jr nz, TEndPlot
cp 96
jp nc, Clip
ld a, (tmpv+1)
ld hl, texture
add a, l
ld l, a
ld a, (tmpu+1)
ld b, a
inc b
ld a, (hl)
TshiftLoop:
rla
djnz TshiftLoop
;push af
ld a, (mask)
ld hl, (pointer)
jr c, TSetPixel
TResPixel:
;ld a, b
cpl
and (hl)
ld (hl), a
jr TEndPlot
TSetPixel:
;ld a, b
or (hl)
ld (hl), a
TEndPlot:
ld hl, mask
rrc (hl)
jr nc, TNoCarry
ld hl, (pointer)
inc hl
ld (pointer), hl
TNoCarry:
ld hl, (tmpu)
ld de, (tmpdu)
add hl, de
ld (tmpu), hl
ld hl, (tmpv)
ld de, (tmpdv)
add hl, de
ld (tmpv), hl
ld a, (temp+1)
sub 50 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld b, a
ld a, (temp)
sub 50 ;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;;
ld hl, temp
inc (hl)
cp b
jr nz, TPlotLoop
bit 0, (IY)
jr nz, aaaa
res 1, (IY)
aaaa:
Clip:
ld hl,(tx1)
ld de, (dx1)
ld a, d
rla
sbc a, a
ld b, a
add hl, de
ld (tx1), hl
ld a, (tx1+2)
adc a, b
ld (tx1+2), a
ld hl,(tx2)
ld de, (dx3)
ld a, d
rla
sbc a, a
ld b, a
add hl, de
ld (tx2), hl
ld a, (tx2+2)
adc a, b
ld (tx2+2), a
ld hl, (tu1)
ld de, (du1)
add hl, de
ld (tu1), hl
ld hl, (tu2)
ld de, (du3)
add hl, de
ld (tu2), hl
ld hl, (tv1)
ld de, (dv1)
add hl, de
ld (tv1), hl
ld hl, (tv2)
ld de, (dv3)
add hl, de
ld (tv2), hl
ld hl, (_ty)
inc hl
ld (_ty), hl
ld de, (y2)
cpHLDE
jp c, TDrawLoop
bit 0, (IY)
jr nz, _TEnd
__TEndLoop:
;Begin tweede keer tekenen:
ld hl, (y2)
ld (_ty), hl
ld hl, (y3)
ld (y2), hl
ld hl, (dx2)
ld (dx1), hl
ld hl, (du2)
ld (du1), hl
ld hl, (dv2)
ld (dv1), hl
ld hl, (x2)
bit 7, h
jr nz, TPos4
ld (tx1+1),hl \ ld a, $FF \ ld (tx1),a
jr TEnd4
TPos4:
ld (tx1+1),hl \ xor a \ ld (tx1),a
Tend4:
ld a, (u2)
ld h, a
ld l, 0
ld (tu1), hl
ld a, (v2)
ld h, a
ld l, 0
ld (tv1), hl
set 0, (IY)
jp TDrawLoop
_TEnd:
ret