Yes, but only the tanks on higher levels will use it, as in the original the tanks get progressively smarter. I keep track of player velocity already, so it would be relatively simple to do so. I would just have to find some point (in the reflected space) where the speed of the shell times the distance to the tank is equal to the speed of the player times the distance from said point to the player.
» Forum
> Your Projects
I've been pretty busy lately, and I gave priority to my serial driver when I did have time to work on projects. I have a paper due tomorrow, (yay procrastination! I need to get back to work.) so hopefully I will have time to work on it this weekend.
I may have made progress since the last update in this thread, but I forgot what exactly I changed since then. I'll check git diffs tomorrow and if it's something impressive I'll upload a screenshot. I think I was working on finding a way to have tanks shoot towards the player's future position, but I'm not sure.
I may have made progress since the last update in this thread, but I forgot what exactly I changed since then. I'll check git diffs tomorrow and if it's something impressive I'll upload a screenshot. I think I was working on finding a way to have tanks shoot towards the player's future position, but I'm not sure.
Very nice! Can’t wait to see what’s coming!
Oh, like predictive aiming? That sounds cool, but maybe also annoying
Did the Wii have that?
commandblockguy wrote:
I think I was working on finding a way to have tanks shoot towards the player's future position, but I'm not sure.
Oh, like predictive aiming? That sounds cool, but maybe also annoying

Some of the later tanks, especially the stationary turrets that bounced shots off the walls, use predictive aiming.
Without it, you could skirt any attack by moving in a straight line, which would make the wide open levels way easier.
Without it, you could skirt any attack by moving in a straight line, which would make the wide open levels way easier.
I'd like to consider this as still under development, but realistically speaking I haven't done anything with it in several months. I'll move it up on my priorities list, and if I do work on it I'll be sure to let you know. Currently the graphics are really lacking, so any help with sprites would be greatly appreciated. That being said, there's no reason to work on the graphics if I'm never going to finish the code.
I'd be happy to help you out with sprites again. This game was a lot of fun on the Wii, and I'd really like to see Tanks! CE be finished. Let me know what kind of sprites you need.
Past projects: HailStorm CE | Contagion CE | HUE CE | VYSION CE
Current projects: VYSION 2 CE | Crossroads CE
Current projects: VYSION 2 CE | Crossroads CE

Almost a year after the last commit, I've decided to start working on this project again.
It's now extremely obvious to me how terrible all of my code was. Bless me, Father, for I have sinned... I was using 18.6 fixed-point numbers on a system where there's no hardware support for 24-bit bitshifts. All of my types are capitalized, and none end in _t. There are random bugs caused by macro arguments not being enclosed in parenthesis, causing issues all over the place, and I was returning pointers to local variables.
I think that's actually a good sign, as it's allowed me to see how I've advanced as a programmer. I hope that I'll be able to see a similar difference with my current code next year.
Anyways, I've started rewriting some of the most poorly written parts of it, and it's now mostly working and compiling on LLVM. The only feature that's currently broken is AI targeting, as my atan2 function used some floating-point numbers that LLVM doesn't like. I'll rewrite it soon so that it only uses integers, which will hopefully also make it much faster, allowing me to make the AI seem smarter (or at the very least, not completely blind) by checking reflections more frequently.
I still haven't completely evaluated what still needs to be done for this project, but my current roadmap is to fix the AI and trig functions as soon as possible, then work out the rest of the physics. I plan to work on the graphics after I finish the physics and controls, as they will make the debugging process for those more complicated. If anyone wants to work on the sprites, though, I imagine that could be done in parallel. I want the final game to have an isometric perspective, so if anyone is interested in helping with sprites, let me know, and I can explain what exactly I need.
Also, now that I have more experience with USB devices, I realize that it might actually be somewhat possible to pair with a Wii Remote with Bluetooth.
Also, I've already written a library for HID devices such as mice and keyboards, which should work as soon as usbdrvce supports interrupt transfers, so I'll definitely add support for those once the USB libs are released. This won't be the "default" gameplay style, of course - I'll still spend time making sure that the keypad controls are easy to use.
It's now extremely obvious to me how terrible all of my code was. Bless me, Father, for I have sinned... I was using 18.6 fixed-point numbers on a system where there's no hardware support for 24-bit bitshifts. All of my types are capitalized, and none end in _t. There are random bugs caused by macro arguments not being enclosed in parenthesis, causing issues all over the place, and I was returning pointers to local variables.
I think that's actually a good sign, as it's allowed me to see how I've advanced as a programmer. I hope that I'll be able to see a similar difference with my current code next year.
Anyways, I've started rewriting some of the most poorly written parts of it, and it's now mostly working and compiling on LLVM. The only feature that's currently broken is AI targeting, as my atan2 function used some floating-point numbers that LLVM doesn't like. I'll rewrite it soon so that it only uses integers, which will hopefully also make it much faster, allowing me to make the AI seem smarter (or at the very least, not completely blind) by checking reflections more frequently.
I still haven't completely evaluated what still needs to be done for this project, but my current roadmap is to fix the AI and trig functions as soon as possible, then work out the rest of the physics. I plan to work on the graphics after I finish the physics and controls, as they will make the debugging process for those more complicated. If anyone wants to work on the sprites, though, I imagine that could be done in parallel. I want the final game to have an isometric perspective, so if anyone is interested in helping with sprites, let me know, and I can explain what exactly I need.
Also, now that I have more experience with USB devices, I realize that it might actually be somewhat possible to pair with a Wii Remote with Bluetooth.
Also, I've already written a library for HID devices such as mice and keyboards, which should work as soon as usbdrvce supports interrupt transfers, so I'll definitely add support for those once the USB libs are released. This won't be the "default" gameplay style, of course - I'll still spend time making sure that the keypad controls are easy to use.
Glad to see you are still looking to complete this very promising project - Also glad that you are able to spruce up the code as well
.

I made a minor update today that fixed a bug with collision detection where you could clip through blocks if you were at the corner of three blocks. I rewrote a good bit of the collision detection code for this, and it revealed a bug where a tank's velocity wasn't used or initialized properly. I've fixed both issues in the latest commit.
I'm not really sure how to do the graphics for this project - I want to preserve the original orthographic view, but I'm not sure how to efficiently convert world space to screen space. If I made a square in world space be drawn as a square in screen space, the perspective would be incorrect and everything would look stretched. If I were to make tiles wider than they are tall in world space so that the aspect ratio is the same, then bullets would move faster up and down than left and right relative to the block grid. If I were to convert between screen and world space properly, that would require me to do a division for each foreground object I draw, which seems inefficient. If anyone knows a better method of doing this, I would appreciate the help.
I'm not really sure how to do the graphics for this project - I want to preserve the original orthographic view, but I'm not sure how to efficiently convert world space to screen space. If I made a square in world space be drawn as a square in screen space, the perspective would be incorrect and everything would look stretched. If I were to make tiles wider than they are tall in world space so that the aspect ratio is the same, then bullets would move faster up and down than left and right relative to the block grid. If I were to convert between screen and world space properly, that would require me to do a division for each foreground object I draw, which seems inefficient. If anyone knows a better method of doing this, I would appreciate the help.
I implemented the orthographic perspective:
At the start of each mission (and each time a mine explodes), I generate a tilemap that represents which block texture each 14x5 region of the screen should have. Then, each frame, I only have to display the tilemap, which is much faster than drawing every single tile face and much more memory efficient than storing the entire screen at once.
The tanks' hitboxes appear much shorter than before, and this is because the hitbox only represents the tank's "footprint" on the floor. This also explains why the tank appears to glitch through blocks - collision detection is working properly, but the tank's footprint is being shown through the wall.
The game is now running much more slowly than before - I think this is partially because each tilemap tile is now smaller than they were in the top-down view, and partially because the game has to do a division for each tank each frame when converting to screen space. I can probably fix the first issue by using partial redraw rather than redrawing the entire tilemap every single frame. If the game still isn't fast enough, I can probably optimize the divisions using a lookup table.
The floor looks really weird to me. I think that part of that is because it has zero detail whatsoever, so it's hard to tell which tiles line up with other tiles. It probably also looks weird because there are no shadows on the floor, while the sides of tiles all have shadows on them. I could probably add a few more tiles to the tileset that are like regular floor tiles but with shadows on them to fix this.

At the start of each mission (and each time a mine explodes), I generate a tilemap that represents which block texture each 14x5 region of the screen should have. Then, each frame, I only have to display the tilemap, which is much faster than drawing every single tile face and much more memory efficient than storing the entire screen at once.
The tanks' hitboxes appear much shorter than before, and this is because the hitbox only represents the tank's "footprint" on the floor. This also explains why the tank appears to glitch through blocks - collision detection is working properly, but the tank's footprint is being shown through the wall.
The game is now running much more slowly than before - I think this is partially because each tilemap tile is now smaller than they were in the top-down view, and partially because the game has to do a division for each tank each frame when converting to screen space. I can probably fix the first issue by using partial redraw rather than redrawing the entire tilemap every single frame. If the game still isn't fast enough, I can probably optimize the divisions using a lookup table.
The floor looks really weird to me. I think that part of that is because it has zero detail whatsoever, so it's hard to tell which tiles line up with other tiles. It probably also looks weird because there are no shadows on the floor, while the sides of tiles all have shadows on them. I could probably add a few more tiles to the tileset that are like regular floor tiles but with shadows on them to fix this.
Another progress report:
I can now also claim my game is 3D, and still be technically correct. The tank sprites are rendered in Blender, using the same orthographic projection as the tiles.
I currently don't have enemy sprites, as I haven't figured out a decent way to use dynamic palettes with Blender. It's anti-aliasing the line between the wooden section of the tank and the colored section, which makes it hard to adjust the colors after it's already rendered. I might have to render each section separately, then merge them later using a mask.
There's still a few other issues with this - the turret's sprite can be quite far off due to a rounding error, and the tank is currently drawn on top of tiles even when that doesn't make sense with perspective. The sprite also doesn't correspond exactly to the hitbox of the tank, which is proving hard to fix. Blender does not provide an easy way to ensure that an object is a certain size in the output image, as far as I can tell.
EDIT: stuff is now being drawn in the correct order
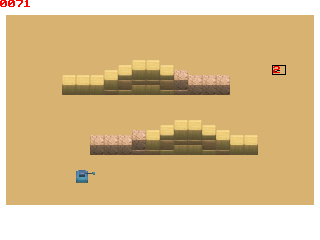
I can now also claim my game is 3D, and still be technically correct. The tank sprites are rendered in Blender, using the same orthographic projection as the tiles.
I currently don't have enemy sprites, as I haven't figured out a decent way to use dynamic palettes with Blender. It's anti-aliasing the line between the wooden section of the tank and the colored section, which makes it hard to adjust the colors after it's already rendered. I might have to render each section separately, then merge them later using a mask.
There's still a few other issues with this - the turret's sprite can be quite far off due to a rounding error, and the tank is currently drawn on top of tiles even when that doesn't make sense with perspective. The sprite also doesn't correspond exactly to the hitbox of the tank, which is proving hard to fix. Blender does not provide an easy way to ensure that an object is a certain size in the output image, as far as I can tell.
EDIT: stuff is now being drawn in the correct order
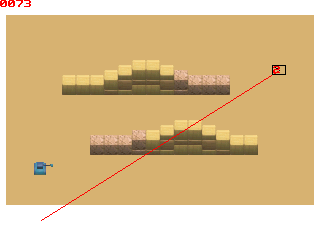
The tank sprite looks great! 3D + base movement makes it look very polished.
Rendering by depth correctly also seems to be running nicely.
Rendering by depth correctly also seems to be running nicely.
Looks good! A few suggestions:
-I'm not sure how you're handling the turret rotation right now, but I'm fairly certain that it works based on clicking a button to rotate the turret in increments. I don't think this is a very good control scheme. Instead, I would suggest: arrow keys for movement, 2nd for rotate turret left, mode for rotate turret right, alpha for fire, and xton for mines. This would make the controls very easy to use and reach, and make the actual feel of gameplay a lot better, I think (also would allow for simultaneous movement/ aiming).
-a floor texture, I suggest pulling from the real game at half-res or just tiling one texture
-a better UI (I know this is a test build, so fairly low priority for now)
-I'm not sure how you're handling the turret rotation right now, but I'm fairly certain that it works based on clicking a button to rotate the turret in increments. I don't think this is a very good control scheme. Instead, I would suggest: arrow keys for movement, 2nd for rotate turret left, mode for rotate turret right, alpha for fire, and xton for mines. This would make the controls very easy to use and reach, and make the actual feel of gameplay a lot better, I think (also would allow for simultaneous movement/ aiming).
-a floor texture, I suggest pulling from the real game at half-res or just tiling one texture
-a better UI (I know this is a test build, so fairly low priority for now)
Past projects: HailStorm CE | Contagion CE | HUE CE | VYSION CE
Current projects: VYSION 2 CE | Crossroads CE
Current projects: VYSION 2 CE | Crossroads CE

Right now the controls are:
Arrow keys to move the tank - each key moves in that absolute direction, rather than the left and right keys turning
2nd fires a shell
Alpha lays a mine
mode and XT0n rotate the barrel
The barrel turning is actually smooth, it's just that I only have 16 sprites for it, so it only picks an approximate sprite to display a more precise barrel direction. I can probably fix that by just doubling the number of sprites, at the expense of program size. I could probably also remove the barrel from the sprite, and use a gfx_Line for that.
I definitely do need a floor texture. I'd prefer one that's tiled, as then I can add shadows to the tiles as part of the tilemap. But I also think that tiling it so that it repeats on the block grid would be pretty boring, so I'm considering doing something similar to ASCII box art and adding a few tile textures with edges that can be tiled to create floorboards of different lengths. I'm not very big on pixel art, though, so if anyone wants to help with that I'd appreciate it. The tiles are 14 wide by 5 tall
And yeah, I haven't even touched the in-game UI yet, aside from the mission start screen and the summary screen at the end. I don't want to have to rework the placement of everything if I decide to move the view over a bit.
Arrow keys to move the tank - each key moves in that absolute direction, rather than the left and right keys turning
2nd fires a shell
Alpha lays a mine
mode and XT0n rotate the barrel
The barrel turning is actually smooth, it's just that I only have 16 sprites for it, so it only picks an approximate sprite to display a more precise barrel direction. I can probably fix that by just doubling the number of sprites, at the expense of program size. I could probably also remove the barrel from the sprite, and use a gfx_Line for that.
I definitely do need a floor texture. I'd prefer one that's tiled, as then I can add shadows to the tiles as part of the tilemap. But I also think that tiling it so that it repeats on the block grid would be pretty boring, so I'm considering doing something similar to ASCII box art and adding a few tile textures with edges that can be tiled to create floorboards of different lengths. I'm not very big on pixel art, though, so if anyone wants to help with that I'd appreciate it. The tiles are 14 wide by 5 tall
And yeah, I haven't even touched the in-game UI yet, aside from the mission start screen and the summary screen at the end. I don't want to have to rework the placement of everything if I decide to move the view over a bit.
Looking great! Is the head of the tank part of your barrel sprite? If not, I definitely think using a gfx_Line for the barrel would be the better option (plus you can probably draw the tip of the barrel without it slowing down too much I would think). Also, are you planning on adding the tread marks at all?
The tread marks are currently quite low priority for me. Currently, I'm redrawing the entire screen each frame, so the tread marks would be overwritten constantly. However, I eventually plan to only do partial redraw, which will not only be faster but also allow for tread marks. Right now I'm focusing on getting functionality working before optimizing things further, so it might be a while before that happens.
Decided to revisit this again, here are today's results:
The red counter in the left is the number of milliseconds per frame. It literally cannot go lower than 16, as that's the rate at which the LCD refreshes. The game's running twice as fast as it's supposed to be, as I forgot to add framerate limiting code, so I'm playing the game at double speed.
The massive speed boost over the last screenshot comes from partial redraw. Basically, I only redraw the parts of the screen that change since the previous frame, instead of drawing every tile on the screen each frame. It's pretty easy to do when using gfx_Blit, but I saw that that was eating up 11ms and decided it was still too slow. I'm now using a system where I malloc a sprite to store the background behind anything that's going to be drawn to the screen, and save it for one frame so that I can redraw on the original buffer.
I also wrote a neat little profiler, so I can tell which parts of the program need to be optimized the most. Here's a report it generated on the third level:
"tilemap" is the full redraw, which only happens when you first start the game, die, or blow something up with a mine. This didn't happen at all within the last 256 frames, which is why it shows as 0.
"undraw" is the function I wrote for the partial redraw, which replaces all of the sprites previously drawn with the old background.
I haven't implemented AI movement yet (still trying to decide between A* and manually annotating different nodes on each level), so it makes sense that that's not taking any time.
"ai_fire" is actually mostly the "stupid" tank, rather than the ones that actively target you.
There are no shells or mines on the screen right now, either.
I'm actually pretty surprised by the amount of time "input" is taking. At first, I thought that the average was being affected by the previous profiler report, which takes place in the middle of the input check and involves doing a bunch of string manipulation. However, I checked the single-frame time, and it was 1ms there as well. I guess that kb_Scan is blocking, so I should either change the keypad mode, write my own function that starts a scan earlier than I need the keypresses, or just decide that the extra 1ms isn't worth it and optimize other things.
Anyways, I think the common factor here is that the things using the most time all involve converting from world-space to screen-space, or world-space to a particular tile. All of those currently involve a division step, which is slow. I'll probably change the unit system so that world space units are exactly 1/256 of a tile, and then use a lookup table to convert the lower 8 bits of the world space position into a sub-tile offset. That way, converting from world-space to a tile is a single byte of assembly (if the input and output are formatted in a certain way), and converting from world-space to screen space is an addition/lookup/multiplication/addition rather than a full division.

The red counter in the left is the number of milliseconds per frame. It literally cannot go lower than 16, as that's the rate at which the LCD refreshes. The game's running twice as fast as it's supposed to be, as I forgot to add framerate limiting code, so I'm playing the game at double speed.
The massive speed boost over the last screenshot comes from partial redraw. Basically, I only redraw the parts of the screen that change since the previous frame, instead of drawing every tile on the screen each frame. It's pretty easy to do when using gfx_Blit, but I saw that that was eating up 11ms and decided it was still too slow. I'm now using a system where I malloc a sprite to store the background behind anything that's going to be drawn to the screen, and save it for one frame so that I can redraw on the original buffer.
I also wrote a neat little profiler, so I can tell which parts of the program need to be optimized the most. Here's a report it generated on the third level:
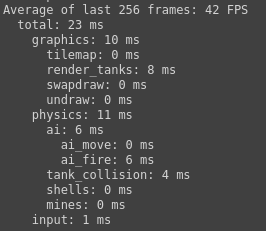
"tilemap" is the full redraw, which only happens when you first start the game, die, or blow something up with a mine. This didn't happen at all within the last 256 frames, which is why it shows as 0.
"undraw" is the function I wrote for the partial redraw, which replaces all of the sprites previously drawn with the old background.
I haven't implemented AI movement yet (still trying to decide between A* and manually annotating different nodes on each level), so it makes sense that that's not taking any time.
"ai_fire" is actually mostly the "stupid" tank, rather than the ones that actively target you.
There are no shells or mines on the screen right now, either.
I'm actually pretty surprised by the amount of time "input" is taking. At first, I thought that the average was being affected by the previous profiler report, which takes place in the middle of the input check and involves doing a bunch of string manipulation. However, I checked the single-frame time, and it was 1ms there as well. I guess that kb_Scan is blocking, so I should either change the keypad mode, write my own function that starts a scan earlier than I need the keypresses, or just decide that the extra 1ms isn't worth it and optimize other things.
Anyways, I think the common factor here is that the things using the most time all involve converting from world-space to screen-space, or world-space to a particular tile. All of those currently involve a division step, which is slow. I'll probably change the unit system so that world space units are exactly 1/256 of a tile, and then use a lookup table to convert the lower 8 bits of the world space position into a sub-tile offset. That way, converting from world-space to a tile is a single byte of assembly (if the input and output are formatted in a certain way), and converting from world-space to screen space is an addition/lookup/multiplication/addition rather than a full division.
Register to Join the Conversation
Have your own thoughts to add to this or any other topic? Want to ask a question, offer a suggestion, share your own programs and projects, upload a file to the file archives, get help with calculator and computer programming, or simply chat with like-minded coders and tech and calculator enthusiasts via the site-wide AJAX SAX widget? Registration for a free Cemetech account only takes a minute.
» Go to Registration page
» Go to Registration page
» Goto page Previous 1, 2, 3, 4 Next
» View previous topic :: View next topic
» View previous topic :: View next topic
Page 2 of 4
» All times are UTC - 5 Hours
You cannot post new topics in this forum
You cannot reply to topics in this forum
You cannot edit your posts in this forum
You cannot delete your posts in this forum
You cannot vote in polls in this forum
You cannot reply to topics in this forum
You cannot edit your posts in this forum
You cannot delete your posts in this forum
You cannot vote in polls in this forum
Advertisement