Alright, here we go. For this example we are going to assume that your choices of color are black, white and grey. After I explain it in this format, you can easily apply it with more shades of grey. My goal is to get this pic:
On my calculator. Also, I want to acieve the least flicker possible so I will use my alternating routine described above. So I will create this pic in Sprite to Hex or however you want to do it, and then when I hit convert it will give me this as an output (note: it's in binary so it's easier for me to convert with my eye):
Code:
The strategy here has been to keep a tally of what we did for the last grey. If we put a one on this layer for the previous grey we encountered, we will put a zero for the current one and vise-versa. This provides us with a checkerboard of grey so to speek, which will virtually eliminate flicker when we switch between the two layers. Now with this knowledge we can create an asm program with this flickerless greyscale to prove my point. Here's 5 minutes of programming (and 30 min of debugging - I made one of the % a $ but didn't catch it for a while
).
Code:
Here's a screenie.
Also, you should note that this looks much much better on calc. But pindurti does a decent job with it. Also, if could have alternated my alternating at the bottom if I had more time (to make it even more checkerboard like) but I prefered copy and pasting becuase I'm not a machine (but your program should do it that way.
Now you can clearly see why this is better than a visibly flashing on and off program.
If you still don't believe me, I can make a screenshot of the flicker type, but this whole thing took me about an hour to do.

On my calculator. Also, I want to acieve the least flicker possible so I will use my alternating routine described above. So I will create this pic in Sprite to Hex or however you want to do it, and then when I hit convert it will give me this as an output (note: it's in binary so it's easier for me to convert with my eye):
Code:
Layer1:
.db %11111111,%11111111,%11111111,%11111111
.db %11010101,%01010101,%01010101,%01010101
.db %11000100,%10100100,%10010101,%01010101
.db %11000101,%01001000,%10010010,%00100101
.db %11000001,%01000100,%10001001,%00010011
.db %11000100,%10100010,%00100100,%01001011
.db %11010101,%01010101,%01010101,%01010101
.db %10101001,%01010101,%01010101,%01010101
.db %10101010,%10100100,%10101010,%10101011
.db %10001001,%01010101,%10101010,%10101011
.db %10100100,%10100100,%10101010,%10101011
.db %11010010,%10101000,%10101010,%10101011
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11111111,%11111111,%11111111,%11111111
Layer2:
.db %11111111,%11111111,%11111111,%11111111
.db %10101010,%10101010,%10101010,%10101011
.db %10010001,%01001010,%00101010,%10101011
.db %10010010,%10100010,%00100100,%01001011
.db %10000100,%10100010,%00100100,%01000101
.db %10010001,%01001000,%10001001,%00100101
.db %10101010,%10101010,%10101010,%10101011
.db %10010100,%10101010,%10101010,%10101011
.db %10010101,%01010010,%01010101,%01010101
.db %10000100,%10101010,%01010101,%01010101
.db %10001001,%01010010,%01010101,%01010101
.db %10101001,%01010001,%01010101,%01010101
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %11111111,%11111111,%11111111,%11111111
The strategy here has been to keep a tally of what we did for the last grey. If we put a one on this layer for the previous grey we encountered, we will put a zero for the current one and vise-versa. This provides us with a checkerboard of grey so to speek, which will virtually eliminate flicker when we switch between the two layers. Now with this knowledge we can create an asm program with this flickerless greyscale to prove my point. Here's 5 minutes of programming (and 30 min of debugging - I made one of the % a $ but didn't catch it for a while

Code:
;Author: Patrick Stetter
;Program:
;Date:
#include "ti83plus.inc"
#include "mirage.inc"
.org $9d93
.db $BB,$6D
ret
.db 1
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db %00000000,%00000000
.db "POOP",0
mainloop:
call fastclear
ld hl,Layer1
ld a,32
ld e,16
ld b,a
call putsprite32bit
call ifastcopy
call fastclear
ld hl,Layer2
ld b,32
ld a,b
ld e,16
call putsprite32bit
call ifastcopy
bcall(_getcsc)
cp skenter
jr nz,mainloop
ret
fastclear:
ld hl,PlotsScreen
ld bc,60*12
fastclearloop:
xor a
ld (hl),a
inc hl
dec bc
ld a,c
or b
jr nz,fastclearloop
ret
putsprite32bit:
;hl=address of sprite
;e=y
;a=x
;b=rows
;32pixels per row
push hl
call getplot
pop de
and 7
jr z,aligned
outerloop:
push bc
push af
ld b,a
ex de,hl
ld c,(hl)
inc hl
ex de,hl
xor a
shiftloop:
srl c
rra
djnz shiftloop
inc hl
or (hl)
ld (hl),a
dec hl
ld a,c
or (hl)
ld (hl),a
inc hl
pop af
push af
ld b,a
ex de,hl
ld c,(hl)
inc hl
ex de,hl
xor a
shiftloop1:
srl c
rra
djnz shiftloop1
inc hl
or (hl)
ld (hl),a
dec hl
ld a,c
or (hl)
ld (hl),a
inc hl
pop af
push af
ld b,a
ex de,hl
ld c,(hl)
inc hl
ex de,hl
xor a
shiftloop2:
srl c
rra
djnz shiftloop2
inc hl
or (hl)
ld (hl),a
dec hl
ld a,c
or (hl)
ld (hl),a
inc hl
pop af
push af
ld b,a
ex de,hl
ld c,(hl)
inc hl
ex de,hl
xor a
shiftloop3:
srl c
rra
djnz shiftloop3
inc hl
or (hl)
ld (hl),a
dec hl
ld a,c
or (hl)
ld (hl),a
ld bc,9
add hl,bc
pop af
pop bc
djnz outerloop
ret
aligned:
push bc
ex de,hl
ld a,(hl)
inc hl
ex de,hl
or (hl)
ld (hl),a
inc hl
ex de,hl
ld a,(hl)
inc hl
ex de,hl
or (hl)
ld (hl),a
inc hl
ex de,hl
ld a,(hl)
inc hl
ex de,hl
or (hl)
ld (hl),a
inc hl
ex de,hl
ld a,(hl)
inc hl
ex de,hl
or (hl)
ld (hl),a
inc hl
ld bc,8
add hl,bc
pop bc
djnz aligned
ret
getplot:
ld h,0
ld d,h
ld l,e
add hl,hl
add hl,de
add hl,hl
add hl,hl
ld e,a
srl e
srl e
srl e
add hl,de
ld de,plotsScreen
add hl,de
ret
Layer1:
.db %11111111,%11111111,%11111111,%11111111
.db %11010101,%01010101,%01010101,%01010101
.db %11000100,%10100100,%10010101,%01010101
.db %11000101,%01001000,%10010010,%00100101
.db %11000001,%01000100,%10001001,%00010011
.db %11000100,%10100010,%00100100,%01001011
.db %11010101,%01010101,%01010101,%01010101
.db %10101001,%01010101,%01010101,%01010101
.db %10101010,%10100100,%10101010,%10101011
.db %10001001,%01010101,%10101010,%10101011
.db %10100100,%10100100,%10101010,%10101011
.db %11010010,%10101000,%10101010,%10101011
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11010101,%01010101,%01010101,%01010101
.db %11111111,%11111111,%11111111,%11111111
Layer2:
.db %11111111,%11111111,%11111111,%11111111
.db %10101010,%10101010,%10101010,%10101011
.db %10010001,%01001010,%00101010,%10101011
.db %10010010,%10100010,%00100100,%01001011
.db %10000100,%10100010,%00100100,%01000101
.db %10010001,%01001000,%10001001,%00100101
.db %10101010,%10101010,%10101010,%10101011
.db %10010100,%10101010,%10101010,%10101011
.db %10010101,%01010010,%01010101,%01010101
.db %10000100,%10101010,%01010101,%01010101
.db %10001001,%01010010,%01010101,%01010101
.db %10101001,%01010001,%01010101,%01010101
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %10101010,%10101010,%10101010,%10101011
.db %11111111,%11111111,%11111111,%11111111
.end
end
Here's a screenie.
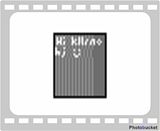
Also, you should note that this looks much much better on calc. But pindurti does a decent job with it. Also, if could have alternated my alternating at the bottom if I had more time (to make it even more checkerboard like) but I prefered copy and pasting becuase I'm not a machine (but your program should do it that way.
Now you can clearly see why this is better than a visibly flashing on and off program.

